Javaを使用してExcel出力する方法です。
Apache POIをインポート
Mavenプロジェクトの場合は、pom.xmlに以下を追記
※poi-ooxmlは2007年以降のExcelを読み込むために必要
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi</artifactId>
<version>5.3.0</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml -->
<dependency>
<groupId>org.apache.poi</groupId>
<artifactId>poi-ooxml</artifactId>
<version>5.3.0</version>
</dependency>
Gradleプロジェクトの場合は、build.gradleに以下を追記
// https://mvnrepository.com/artifact/org.apache.poi/poi
implementation group: 'org.apache.poi', name: 'poi', version: '5.3.0'
// https://mvnrepository.com/artifact/org.apache.poi/poi-ooxml
implementation group: 'org.apache.poi', name: 'poi-ooxml', version: '5.3.0'
実装
1.以下のようにコードを記載
package jp.example;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExportExcel {
public static void main(String[] args) {
Workbook newWorkbook = null;
try {
// Excelを作成
newWorkbook = new XSSFWorkbook();
// シートを作成
Sheet outputSheet = newWorkbook.createSheet("sample");
// 行を作成(0がExcelの1行目)
Row outputRow0 = outputSheet.createRow(0);
// セルを作成(0がExcelの1列目)
Cell outputCell_header_no = outputRow0.createCell(0);
Cell outputCell_header_product = outputRow0.createCell(1);
Cell outputCell_header_price = outputRow0.createCell(2);
// セルに値を設定
outputCell_header_no.setCellValue("項番");
outputCell_header_product.setCellValue("商品");
outputCell_header_price.setCellValue("値段");
// 行を作成
Row outputRow1 = outputSheet.createRow(1);
// セルを作成
Cell outputCell_no_1 = outputRow1.createCell(0);
Cell outputCell_product_1 = outputRow1.createCell(1);
Cell outputCell_price_1 = outputRow1.createCell(2);
// セルに値を設定
outputCell_no_1.setCellValue("1");
outputCell_product_1.setCellValue("りんご");
outputCell_price_1.setCellValue("200円");
// 出力用のストリームを用意
FileOutputStream out = null;
out = new FileOutputStream("C:\\tmp\\出力先.xlsx");
// ファイルへ出力
newWorkbook.write(out);
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
// TODO 自動生成された catch ブロック
e.printStackTrace();
} finally {
try {
if (newWorkbook != null) {
newWorkbook.close();
}
} catch (IOException e) {
// TODO 自動生成された catch ブロック
e.printStackTrace();
}
}
}
}
2.実行すると、指定したパスにエクセルが作成
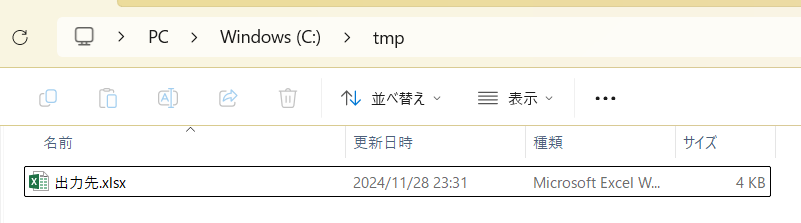
3.中身はコードで実装した通りになっている
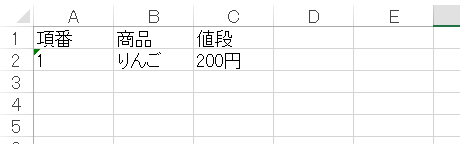
コメント