【Unity】ある範囲内でランダムな場所にGameObjectを生成させる方法
ある範囲内でランダムな場所にGameObjectを生成させる方法を紹介します。
対角線上に空のゲームオブジェクトを設置して、その範囲内にGameObjectを生成させるようにします。
目次
完成動作
※生成スピードを1秒に1個ぐらいにしてます。
やり方(動画)
やり方(ブログ)
ランダムに生成させたい範囲の対角線上に空のGameObjectを設置
1."Create Empty"をクリックして空のGameObjectを作成
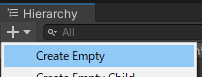
2.名前を"RangeA"とする。
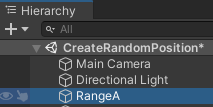
3.同様に"Create Empty"を作成して名前を"RangeB"とする。
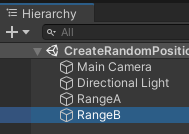
4.RangeAとRangeBのゲームオブジェクトをランダムに生成させたい範囲の対角線上に設置する。
今回は例として以下の位置にした。
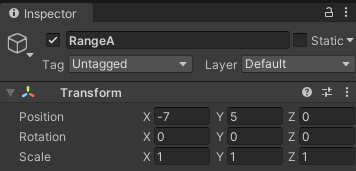
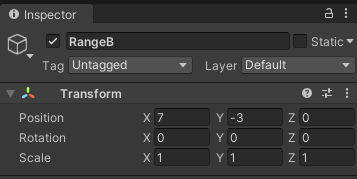
指定した範囲内でランダムな場所にGameObjectを生成するスクリプトを作成
1.CreateRandomPosition.csという名前のスクリプトを作成して、以下のコードを記載する。
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CreateRangeRandomPosition : MonoBehaviour
{
[SerializeField]
[Tooltip("生成するGameObject")]
private GameObject createPrefab;
[SerializeField]
[Tooltip("生成する範囲A")]
private Transform rangeA;
[SerializeField]
[Tooltip("生成する範囲B")]
private Transform rangeB;
// 経過時間
private float time;
// Update is called once per frame
void Update()
{
// 前フレームからの時間を加算していく
time = time + Time.deltaTime;
// 約1秒置きにランダムに生成されるようにする。
if(time > 1.0f)
{
// rangeAとrangeBのx座標の範囲内でランダムな数値を作成
float x = Random.Range(rangeA.position.x, rangeB.position.x);
// rangeAとrangeBのy座標の範囲内でランダムな数値を作成
float y = Random.Range(rangeA.position.y, rangeB.position.y);
// rangeAとrangeBのz座標の範囲内でランダムな数値を作成
float z = Random.Range(rangeA.position.z, rangeB.position.z);
// GameObjectを上記で決まったランダムな場所に生成
Instantiate(createPrefab, new Vector3(x,y,z), createPrefab.transform.rotation);
// 経過時間リセット
time = 0f;
}
}
}
スクリプトをアタッチ
1.スクリプトをアタッチする空のGameObjectを作成して名前を"CreateRandomManager"にする。
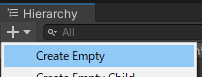
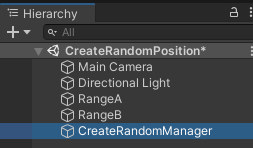
2."CreateRandomManager"にCreateRandomPositionスクリプトをアタッチ
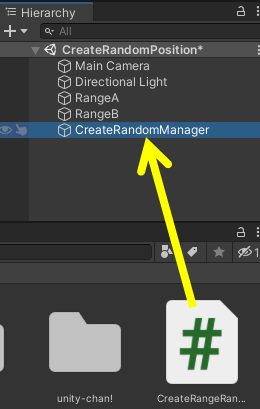
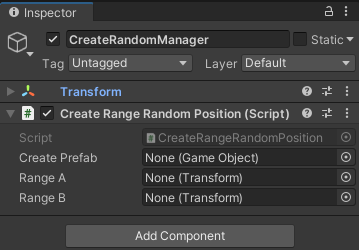
“CreateRandomManager"の各プロパティに値を設定
1."Create Prefab"プロパティに生成したいプレハブを設定(今回は例として事前に作成しておいた赤色のCubeプレハブ)
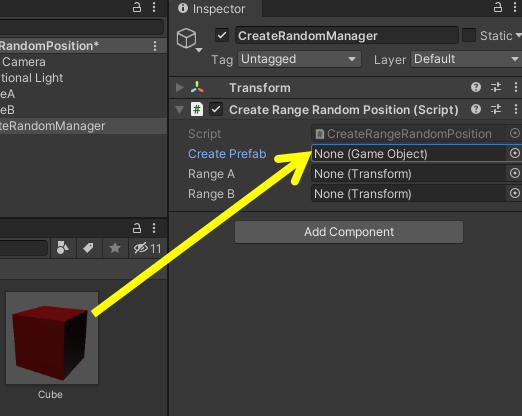
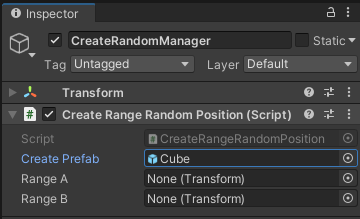
2."Range A"プロパティにRangeAゲームオブジェクトを設定
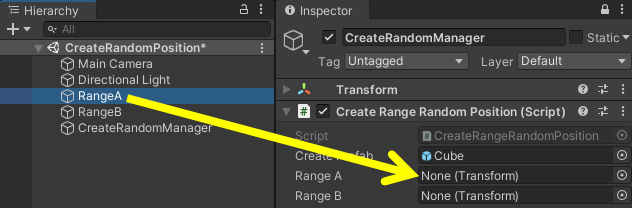
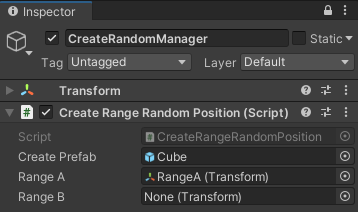
3."Range B"プロパティにRangeBゲームオブジェクトを設定
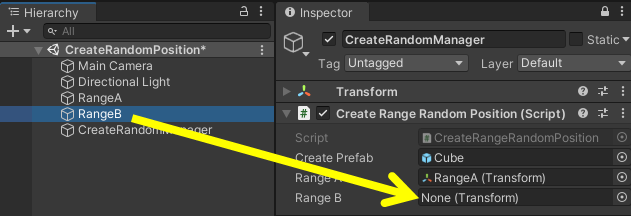
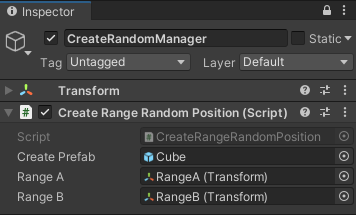
完成
これでゲームをスタートするとRangeAとRangeBの範囲内のランダムな場所にGameObjectが生成されるようになる。
ディスカッション
コメント一覧
まだ、コメントがありません